Popup messages
A Toast provides simple feedback about an operation in a small popup. It only fills the amount of space required for the message and the current activity remains visible and interactive. Toasts automatically disappear after a timeout.
Snackbar provide brief messages about app processes at the bottom of the screen. It does not require user input to disappear. It may have an action button.
Snackbar
Toast
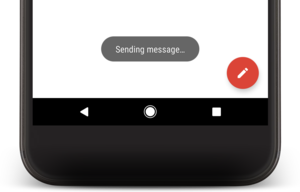
Snackbar
Typically Snackbar is attached to a CoordinatorLayout to gain additional features:
- user can dismiss the Snackbar by swiping it away
- the layout moves some other UI elements when the Snackbar appears like in the video above
If you just want to show a message to the user and won't need to call any of the Snackbar object's utility methods, you don't need to keep the reference to the Snackbar after you call show().
Snackbar.make(
findViewById(R.id.myCoordinatorLayout),
R.string.email_sent,
Snackbar.LENGTH_SHORT
).show()
To add an action to a Snackbar message, you need to define a listener object that implements the View.OnClickListener interface. The system calls your listener's onClick() method if the user clicks on the message action.
class MyUndoListener : View.OnClickListener {
fun onClick(v: View) {
// Code to undo the user's last action
}
}
// ...
val mySnackbar = Snackbar.make(findViewById(R.id.myCoordinatorLayout),
R.string.email_archived, Snackbar.LENGTH_SHORT)
mySnackbar.setAction(R.string.undo_string, MyUndoListener())
mySnackbar.show()
A Snackbar automatically goes away after a short time, so you can't count on the user seeing the message or having a chance to press the button. For this reason, you should consider offering an alternate way to perform any Snackbar action.
Toast
Toast.makeText(context, text, Toast.LENGTH_SHORT).show()
By default TextView is used to show toast message. You can customize it if necessary
val toast = Toast.makeText(this, "Toast message", Toast.LENGTH_LONG).apply {
view!!.setBackgroundColor(Color.MAGENTA)
view!!.findViewById<TextView>(R.id.message).apply {
textSize = 25f
gravity = Gravity.CENTER
setCompoundDrawablesWithIntrinsicBounds(R.mipmap.my_ic_toast, 0,0,0)
compoundDrawablePadding = 16
}
}
toast.show()
Additionally you can set custom view or change a gravity of toast
// getting the View object as defined in the customtoast.xml file
val toastView: ViewGroup = layoutInflater.inflate(
R.layout.customtoast,
findViewById<ViewGroup>(R.id.custom_toast_container)
).apply{
val text = findViewById(R.id.textView) as TextView
text.text = "message"
}
// creating the Toast object
Toast(applicationContext).apply {
duration = Toast.LENGTH_SHORT
setGravity(Gravity.CENTER_VERTICAL, 0, 0) // show in center of screen
// deprecated since API 30+, apps that are in the background will
// not have custom toast views displayed.
setView(toastView) //setting the view of custom toast layout
}.show()
Toast vs Snackbar
The Snackbar class supersedes Toast. While Toast is currently still supported, Snackbar is now the preferred way to display brief, transient messages to the user.
Toast:
- Api level 1
- activity not required, can be shown on Android home or above other apps
- only show message, user can't perform any action
- it can't be dismissed by swiping
Snackbar:
- API Level 23
- activity required
- possible to add action, for example "undo"
- can be dismissed by swiping